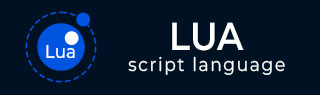
- Lua Tutorial
- Lua - Home
- Lua Basics
- Lua - Overview
- Lua - Environment
- Lua - Basic Syntax
- Lua - Comments
- Lua - Print Hello World
- Lua - Variables
- Lua - Data Types
- Lua - Operators
- Lua - Loops
- Lua - Generic For
- Lua - Decision Making
- Lua - Date and Time
- Lua Functions
- Lua - Functions
- Lua - Multiple Results
- Lua - Named Arguments
- Lua - Default/Optional Arguments
- Lua - Closures
- Lua - Uses of Closures
- Lua - Local Functions
- Lua - Anonymous Functions
- Lua - Functions in Table
- Lua - Proper Tail Calls
- Lua Strings
- Lua - Strings
- Lua - String Concatenation
- Lua - Loop Through String
- Lua - String to Int
- Lua - Split String
- Lua - Check String is NULL
- Lua Arrays
- Lua - Arrays
- Lua - Multi-dimensional Arrays
- Lua - Array Length
- Lua - Iterating Over Arrays
- Lua - Slicing Arrays
- Lua - Sorting Arrays
- Lua - Merging Arrays
- Lua - Sparse Arrays
- Lua - Searching Arrays
- Lua - Resizing Arrays
- Lua - Array to String Conversion
- Lua - Array as Stack
- Lua - Array as Queue
- Lua - Array with Metatables
- Lua - Immutable Arrays
- Lua - Shuffling Arrays
- Lua Iterators
- Lua - Iterators
- Lua - Stateless Iterators
- Lua - Stateful Iterators
- Lua - Built-in Iterators
- Lua - Custom Iterators
- Lua - Iterator Closures
- Lua - Infinite Iterators
- Lua - File Iterators
- Lua - Table Iterators
- Lua - Numeric Iterators
- Lua - Reverse Iterators
- Lua - Filter Iterators
- Lua - Range Iterators
- Lua - Chaining Iterators
- Lua Tables
- Lua - Tables
- Lua - Tables as Arrays
- Lua - Tables as Dictionaries
- Lua - Tables as Sets
- Lua - Table Length
- Lua - Table Iteration
- Lua - Table Constructors
- Lua - Loop through Table
- Lua - Merge Tables
- Lua - Nested Tables
- Lua - Accessing Table Fields
- Lua - Copy Table by Value
- Lua - Get Entries from Table
- Lua - Table Metatables
- Lua - Tables as Objects
- Lua - Table Inheritance
- Lua - Table Cloning
- Lua - Table Sorting
- Lua - Table Searching
- Lua - Table Serialization
- Lua - Weak Tables
- Lua - Table Memory Management
- Lua - Tables as Stacks
- Lua - Tables as Queues
- Lua - Sparse Tables
- Lua Lists
- Lua - Lists
- Lua - Inserting Elements into Lists
- Lua - Removing Elements from Lists
- Lua - Iterating Over Lists
- Lua - Reverse Iterating Over Lists
- Lua - Accessing List Elements
- Lua - Modifying List Elements
- Lua - List Length
- Lua - Concatenate Lists
- Lua - Slicing Lists
- Lua - Sorting Lists
- Lua - Reversing Lists
- Lua - Searching in Lists
- Lua - Shuffling List
- Lua - Multi-dimensional Lists
- Lua - Sparse Lists
- Lua - Lists as Stacks
- Lua - Lists as Queues
- Lua - Functional Operations on Lists
- Lua - Immutable Lists
- Lua - List Serialization
- Lua - Metatables with Lists
- Lua Modules
- Lua - Modules
- Lua - Returning Functions from Modules
- Lua - Returning Functions Table from Modules
- Lua - Module Scope
- Lua - SubModule
- Lua - Module Caching
- Lua - Custom Module Loaders
- Lua - Namespaces
- Lua - Singleton Modules
- Lua - Sharing State Between Modules
- Lua - Module Versioning
- Lua Metatables
- Lua - Metatables
- Lua - Chaining Metatables
- Lua - Proxy Tables with Metatables
- Lua - Use Cases for Proxy Table
- Lua - Delegation and Tracing via Proxy Tables
- Lua - Metatables vs Metamethods
- Lua - Fallback Mechanisms in Metatables
- Lua - Fallback Cases for Indexing Metamethods
- Lua - Fallback Cases for Arithmetic and Comparison Metamethods
- Lua - Fallback Cases for Other Metamethods
- Lua - Customizing Behavior with Metatables
- Lua - Controlling Table Access
- Lua - Overloading Operators
- Lua - Customizing Comparisons
- Lua - Making a Table Callable
- Lua - Customizing String Representation
- Lua - Controlling Metatable Access
- Lua Coroutines
- Lua - Coroutines
- Lua - Coroutine Lifecycle
- Lua - Communication Between Coroutines
- Lua - Coroutines vs Threads
- Lua - Chaining Coroutines
- Lua - Chaining Coroutines With Scheduler
- Lua - Chaining Coroutines Using Queues
- Lua - Coroutine Control Flow
- Lua - Nested Coroutines
- Lua File Handling
- Lua - File I/O
- Lua - Opening Files
- Lua - Modes for File Access
- Lua - Reading Files
- Lua - Writing Files
- Lua - Closing Files
- Lua - Renaming Files
- Lua - Deleting Files
- Lua - File Buffers and Flushing
- Lua - Reading Files Line by Line
- Lua - Binary File Handling
- Lua - File Positioning
- Lua - Appending to Files
- Lua - Error Handling in File Operations
- Lua - Checking if File exists
- Lua - Checking if File is Readable
- Lua - Checking if File is Writable
- Lua - Checking if File is ReadOnly
- Lua - File Descriptors
- Lua - Creating Temporary Files
- Lua - File Iterators
- Lua - Working with Large Files
- Lua Advanced
- Lua - Error Handling
- Lua - Debugging
- Lua - Garbage Collection
- Lua - Object Oriented
- Lua - Web Programming
- Lua - Database Access
- Lua - Game Programing
- Sorting Algorithms
- Lua - Bubble Sort
- Lua - Insertion Sort
- Lua - Selection Sort
- Lua - Merge Sort
- Lua - Quick Sort
- Searching Algorithms
- Lua - Linear Search
- Lua - Binary Search
- Lua - Jump Search
- Lua - Interpolation Search
- Regular Expression
- Lua - Pattern Matching
- Lua - string.find() method
- Lua - string.gmatch() method
- Lua - string.gsub() method
- Lua Useful Resources
- Lua - Quick Guide
- Lua - Useful Resources
- Lua - Discussion
Lua - Custom Iterators
Iterators in Lua are functions which helps in traversing or iterating a collection. We can write our own custom iterator easily. Consider the following code to write a iterator which can be used in a for loop to generate a sequence of square of numbers.
Example - a Custom stateless Iterator
Following is an example of a custom stateless iterator to get a sequence of square of numbers.
main.lua
-- define an iterator to get square of an element -- in case index >= state, lua will return Nil and terminates the iteration function square(state,index) -- if index is less than maximum number if index < state then -- increment the index index = index + 1 return index, index*index end end -- define an iterator function squares(value) -- iterator, state, initial value return square, value, 0 end -- call the iterator to get sequence of 5 numbers and their squares for index, value in squares(5) do print(index, value) end
Output
When we run the above program, we will get the following output−
1 1 2 4 3 9 4 16 5 25
Example - a Custom Stateful Iterator
Following is an example of a custom stateful iterator which accepts an array and return squared value.
main.lua
-- Initialize an array array = {1, 2, 3, 4, 5, 6} -- return a stateful iterator to get values of collection passed function elementIterator (collection) local index = 0 local count = #collection -- The closure function is returned return function () index = index + 1 if index <= count then -- return the current element of the iterator local value = collection[index]; return index, value * value end end end -- loop through the iterator for index, value in elementIterator(array) do -- print the element print(index, value) end
Output
When we run the above program, we will get the following output−
1 1 2 4 3 9 4 16 5 25 6 36
Advertisements