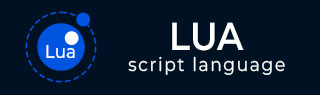
- Lua Tutorial
- Lua - Home
- Lua Basics
- Lua - Overview
- Lua - Environment
- Lua - Basic Syntax
- Lua - Comments
- Lua - Print Hello World
- Lua - Variables
- Lua - Data Types
- Lua - Operators
- Lua - Loops
- Lua - Generic For
- Lua - Decision Making
- Lua - Date and Time
- Lua Functions
- Lua - Functions
- Lua - Multiple Results
- Lua - Named Arguments
- Lua - Default/Optional Arguments
- Lua - Closures
- Lua - Uses of Closures
- Lua - Local Functions
- Lua - Anonymous Functions
- Lua - Functions in Table
- Lua - Proper Tail Calls
- Lua Strings
- Lua - Strings
- Lua - String Concatenation
- Lua - Loop Through String
- Lua - String to Int
- Lua - Split String
- Lua - Check String is NULL
- Lua Arrays
- Lua - Arrays
- Lua - Multi-dimensional Arrays
- Lua - Array Length
- Lua - Iterating Over Arrays
- Lua - Slicing Arrays
- Lua - Sorting Arrays
- Lua - Merging Arrays
- Lua - Sparse Arrays
- Lua - Searching Arrays
- Lua - Resizing Arrays
- Lua - Array to String Conversion
- Lua - Array as Stack
- Lua - Array as Queue
- Lua - Array with Metatables
- Lua - Immutable Arrays
- Lua - Shuffling Arrays
- Lua Iterators
- Lua - Iterators
- Lua - Stateless Iterators
- Lua - Stateful Iterators
- Lua - Built-in Iterators
- Lua - Custom Iterators
- Lua - Iterator Closures
- Lua - Infinite Iterators
- Lua - File Iterators
- Lua - Table Iterators
- Lua - Numeric Iterators
- Lua - Reverse Iterators
- Lua - Filter Iterators
- Lua - Range Iterators
- Lua - Chaining Iterators
- Lua Tables
- Lua - Tables
- Lua - Tables as Arrays
- Lua - Tables as Dictionaries
- Lua - Tables as Sets
- Lua - Table Length
- Lua - Table Iteration
- Lua - Table Constructors
- Lua - Loop through Table
- Lua - Merge Tables
- Lua - Nested Tables
- Lua - Accessing Table Fields
- Lua - Copy Table by Value
- Lua - Get Entries from Table
- Lua - Table Metatables
- Lua - Tables as Objects
- Lua - Table Inheritance
- Lua - Table Cloning
- Lua - Table Sorting
- Lua - Table Searching
- Lua - Table Serialization
- Lua - Weak Tables
- Lua - Table Memory Management
- Lua - Tables as Stacks
- Lua - Tables as Queues
- Lua - Sparse Tables
- Lua Lists
- Lua - Lists
- Lua - Inserting Elements into Lists
- Lua - Removing Elements from Lists
- Lua - Iterating Over Lists
- Lua - Reverse Iterating Over Lists
- Lua - Accessing List Elements
- Lua - Modifying List Elements
- Lua - List Length
- Lua - Concatenate Lists
- Lua - Slicing Lists
- Lua - Sorting Lists
- Lua - Reversing Lists
- Lua - Searching in Lists
- Lua - Shuffling List
- Lua - Multi-dimensional Lists
- Lua - Sparse Lists
- Lua - Lists as Stacks
- Lua - Lists as Queues
- Lua - Functional Operations on Lists
- Lua - Immutable Lists
- Lua - List Serialization
- Lua - Metatables with Lists
- Lua Modules
- Lua - Modules
- Lua - Returning Functions from Modules
- Lua - Returning Functions Table from Modules
- Lua - Module Scope
- Lua - SubModule
- Lua - Module Caching
- Lua - Custom Module Loaders
- Lua - Namespaces
- Lua - Singleton Modules
- Lua - Sharing State Between Modules
- Lua - Module Versioning
- Lua Metatables
- Lua - Metatables
- Lua - Chaining Metatables
- Lua - Proxy Tables with Metatables
- Lua - Use Cases for Proxy Table
- Lua - Delegation and Tracing via Proxy Tables
- Lua - Metatables vs Metamethods
- Lua - Fallback Mechanisms in Metatables
- Lua - Fallback Cases for Indexing Metamethods
- Lua - Fallback Cases for Arithmetic and Comparison Metamethods
- Lua - Fallback Cases for Other Metamethods
- Lua - Customizing Behavior with Metatables
- Lua - Controlling Table Access
- Lua - Overloading Operators
- Lua - Customizing Comparisons
- Lua - Making a Table Callable
- Lua - Customizing String Representation
- Lua - Controlling Metatable Access
- Lua Coroutines
- Lua - Coroutines
- Lua - Coroutine Lifecycle
- Lua - Communication Between Coroutines
- Lua - Coroutines vs Threads
- Lua - Chaining Coroutines
- Lua - Chaining Coroutines With Scheduler
- Lua - Chaining Coroutines Using Queues
- Lua - Coroutine Control Flow
- Lua - Nested Coroutines
- Lua File Handling
- Lua - File I/O
- Lua - Opening Files
- Lua - Modes for File Access
- Lua - Reading Files
- Lua - Writing Files
- Lua - Closing Files
- Lua - Renaming Files
- Lua - Deleting Files
- Lua - File Buffers and Flushing
- Lua - Reading Files Line by Line
- Lua - Binary File Handling
- Lua - File Positioning
- Lua - Appending to Files
- Lua - Error Handling in File Operations
- Lua - Checking if File exists
- Lua - Checking if File is Readable
- Lua - Checking if File is Writable
- Lua - Checking if File is ReadOnly
- Lua - File Descriptors
- Lua - Creating Temporary Files
- Lua - File Iterators
- Lua - Working with Large Files
- Lua Advanced
- Lua - Error Handling
- Lua - Debugging
- Lua - Garbage Collection
- Lua - Object Oriented
- Lua - Web Programming
- Lua - Database Access
- Lua - Game Programing
- Sorting Algorithms
- Lua - Bubble Sort
- Lua - Insertion Sort
- Lua - Selection Sort
- Lua - Merge Sort
- Lua - Quick Sort
- Searching Algorithms
- Lua - Linear Search
- Lua - Binary Search
- Lua - Jump Search
- Lua - Interpolation Search
- Regular Expression
- Lua - Pattern Matching
- Lua - string.find() method
- Lua - string.gmatch() method
- Lua - string.gsub() method
- Lua Useful Resources
- Lua - Quick Guide
- Lua - Useful Resources
- Lua - Discussion
Lua - Nested Tables
Nested Table is a very important concept which can help in storing the structured data in a data-struture. Consider following scenarios−
-
Table of Student Details having student and address as a nested tables.
studentDetails = { student = { name = "Robert", age = "12"}, address = { city = "HYD", pincode = "500031"} }
-
Table of Game with details of player and scores as a nested tables.
playerDetails = { player = { name = "Robert", level = "12"}, scores = { 23, 33, 77, 94, 78} }
and so on..
Accessing nested Table fields
A nested field of a table can be accessed in two ways.
Using array like, [] notation.
Using . notation.
Example - Using array notation to access nested field with text based key.
Consider the following example, where we're accessing a student name using array notation as text based key.
main.lua
-- define a nested structure studentDetails = { student = { name = "Robert", age = "12"}, address = { city = "HYD", pincode = "500031"} } -- access student name name = studentDetails["student"]["name"] -- print student name print(name)
Output
When the above code is built and executed, it produces the following result −
Robert
Example - Using array notation to access nested field with index based key.
Consider another example, where we're accessing a student details using array notation as number based key.
main.lua
-- define a nested structure students = { [1] = { name = "Robert", rollNo = "1"}, [2] = { name = "Julie", rollNo = "2"}, [3] = { name = "Adam", rollNo = "3"}, } -- access student name name = students[2]["name"] -- print student name print(name)
Output
When the above code is built and executed, it produces the following result −
Julie
Example - Navigating through nested Table
We can access a nested array easily and can navigate through it. See the example below:
main.lua
-- define a nested structure playerDetails = { player = { name = "Robert", level = "12"}, scores = { 23, 33, 77, 94, 78} } -- access player name name = playerDetails["player"][name"] -- print player name print(name) -- access player scores scores = playerDetails["scores"] -- print player scores for index, score in ipairs(scores) do print(index, score) end
Output
When the above code is built and executed, it produces the following result −
Robert 1 23 2 33 3 77 4 94 5 78