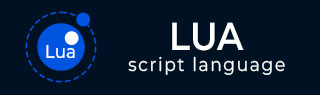
- Lua Tutorial
- Lua - Home
- Lua Basics
- Lua - Overview
- Lua - Environment
- Lua - Basic Syntax
- Lua - Comments
- Lua - Print Hello World
- Lua - Variables
- Lua - Data Types
- Lua - Operators
- Lua - Loops
- Lua - Generic For
- Lua - Decision Making
- Lua - Date and Time
- Lua Functions
- Lua - Functions
- Lua - Multiple Results
- Lua - Named Arguments
- Lua - Default/Optional Arguments
- Lua - Closures
- Lua - Uses of Closures
- Lua - Local Functions
- Lua - Anonymous Functions
- Lua - Functions in Table
- Lua - Proper Tail Calls
- Lua Strings
- Lua - Strings
- Lua - String Concatenation
- Lua - Loop Through String
- Lua - String to Int
- Lua - Split String
- Lua - Check String is NULL
- Lua Arrays
- Lua - Arrays
- Lua - Multi-dimensional Arrays
- Lua - Array Length
- Lua - Iterating Over Arrays
- Lua - Slicing Arrays
- Lua - Sorting Arrays
- Lua - Merging Arrays
- Lua - Sparse Arrays
- Lua - Searching Arrays
- Lua - Resizing Arrays
- Lua - Array to String Conversion
- Lua - Array as Stack
- Lua - Array as Queue
- Lua - Array with Metatables
- Lua - Immutable Arrays
- Lua - Shuffling Arrays
- Lua Iterators
- Lua - Iterators
- Lua - Stateless Iterators
- Lua - Stateful Iterators
- Lua - Built-in Iterators
- Lua - Custom Iterators
- Lua - Iterator Closures
- Lua - Infinite Iterators
- Lua - File Iterators
- Lua - Table Iterators
- Lua - Numeric Iterators
- Lua - Reverse Iterators
- Lua - Filter Iterators
- Lua - Range Iterators
- Lua - Chaining Iterators
- Lua Tables
- Lua - Tables
- Lua - Tables as Arrays
- Lua - Tables as Dictionaries
- Lua - Tables as Sets
- Lua - Table Length
- Lua - Table Iteration
- Lua - Table Constructors
- Lua - Loop through Table
- Lua - Merge Tables
- Lua - Nested Tables
- Lua - Accessing Table Fields
- Lua - Copy Table by Value
- Lua - Get Entries from Table
- Lua - Table Metatables
- Lua - Tables as Objects
- Lua - Table Inheritance
- Lua - Table Cloning
- Lua - Table Sorting
- Lua - Table Searching
- Lua - Table Serialization
- Lua - Weak Tables
- Lua - Table Memory Management
- Lua - Tables as Stacks
- Lua - Tables as Queues
- Lua - Sparse Tables
- Lua Lists
- Lua - Lists
- Lua - Inserting Elements into Lists
- Lua - Removing Elements from Lists
- Lua - Iterating Over Lists
- Lua - Reverse Iterating Over Lists
- Lua - Accessing List Elements
- Lua - Modifying List Elements
- Lua - List Length
- Lua - Concatenate Lists
- Lua - Slicing Lists
- Lua - Sorting Lists
- Lua - Reversing Lists
- Lua - Searching in Lists
- Lua - Shuffling List
- Lua - Multi-dimensional Lists
- Lua - Sparse Lists
- Lua - Lists as Stacks
- Lua - Lists as Queues
- Lua - Functional Operations on Lists
- Lua - Immutable Lists
- Lua - List Serialization
- Lua - Metatables with Lists
- Lua Modules
- Lua - Modules
- Lua - Returning Functions from Modules
- Lua - Returning Functions Table from Modules
- Lua - Module Scope
- Lua - SubModule
- Lua - Module Caching
- Lua - Custom Module Loaders
- Lua - Namespaces
- Lua - Singleton Modules
- Lua - Sharing State Between Modules
- Lua - Module Versioning
- Lua Metatables
- Lua - Metatables
- Lua - Chaining Metatables
- Lua - Proxy Tables with Metatables
- Lua - Use Cases for Proxy Table
- Lua - Delegation and Tracing via Proxy Tables
- Lua - Metatables vs Metamethods
- Lua - Fallback Mechanisms in Metatables
- Lua - Fallback Cases for Indexing Metamethods
- Lua - Fallback Cases for Arithmetic and Comparison Metamethods
- Lua - Fallback Cases for Other Metamethods
- Lua - Customizing Behavior with Metatables
- Lua - Controlling Table Access
- Lua - Overloading Operators
- Lua - Customizing Comparisons
- Lua - Making a Table Callable
- Lua - Customizing String Representation
- Lua - Controlling Metatable Access
- Lua Coroutines
- Lua - Coroutines
- Lua - Coroutine Lifecycle
- Lua - Communication Between Coroutines
- Lua - Coroutines vs Threads
- Lua - Chaining Coroutines
- Lua - Chaining Coroutines With Scheduler
- Lua - Chaining Coroutines Using Queues
- Lua - Coroutine Control Flow
- Lua - Nested Coroutines
- Lua File Handling
- Lua - File I/O
- Lua - Opening Files
- Lua - Modes for File Access
- Lua - Reading Files
- Lua - Writing Files
- Lua - Closing Files
- Lua - Renaming Files
- Lua - Deleting Files
- Lua - File Buffers and Flushing
- Lua - Reading Files Line by Line
- Lua - Binary File Handling
- Lua - File Positioning
- Lua - Appending to Files
- Lua - Error Handling in File Operations
- Lua - Checking if File exists
- Lua - Checking if File is Readable
- Lua - Checking if File is Writable
- Lua - Checking if File is ReadOnly
- Lua - File Descriptors
- Lua - Creating Temporary Files
- Lua - File Iterators
- Lua - Working with Large Files
- Lua Advanced
- Lua - Error Handling
- Lua - Debugging
- Lua - Garbage Collection
- Lua - Object Oriented
- Lua - Web Programming
- Lua - Database Access
- Lua - Game Programing
- Sorting Algorithms
- Lua - Bubble Sort
- Lua - Insertion Sort
- Lua - Selection Sort
- Lua - Merge Sort
- Lua - Quick Sort
- Searching Algorithms
- Lua - Linear Search
- Lua - Binary Search
- Lua - Jump Search
- Lua - Interpolation Search
- Regular Expression
- Lua - Pattern Matching
- Lua - string.find() method
- Lua - string.gmatch() method
- Lua - string.gsub() method
- Lua Useful Resources
- Lua - Quick Guide
- Lua - Useful Resources
- Lua - Discussion
Lua - Print Hello World
This tutorial will teach you how to write a simple Hello World program using Lua Programming language. This program will make use of Lua built-in print() function to print the string.
Hello World Program in Lua
Printing "Hello World" is the first program in Lua. This program will not take any user input, it will just print text on the output screen. It is used to test if the software needed to compile and run the program has been installed correctly.
Steps
The following are the steps to write a Lua program to print Hello World –
- Step 1: Install Lua. Make sure that Lua is installed on your system or not. If Lua is not installed, then install it from here: https://github.com/rjpcomputing/luaforwindows/releases
- Step 2: Choose Text Editor or IDE to write the code.
- Step 3: Open Text Editor or IDE, create a new file, and write the code to print Hello World.
- Step 4: Save the file with a file name and extension ".lua".
- Step 5: Compile/Run the program.
Example - Lua Program to Print Hello World
Consider the below code that will print "Hello World" or any message that you will write inside the print() method on the screen.
main.lua
# Lua code to print "Hello World" print ("Hello World!")
In the above code, we wrote two lines. The first line is the Lua comment that will be ignored by the Lua Compiler, and the second line is the print() statement that will print the given message ("Hello World!") on the output screen.
Output
Hello World!
Different Ways to Write and Execute Hello World Program
Using Lua Interpreter Command Prompt Mode
It is very easy to display the Hello World message using the Lua interpreter. Launch the Lua interpreter installed and issue the print statement from the Lua prompt as follows −
Example - Using Command Line Interpreter
Lua 5.1.5 Copyright (C) 1994-2012 Lua.org, PUC-Rio > print("Hello World!") Hello World! >
Similarly, Hello World message is printed on Linux System.
Example - Using Linux terminal
Lua 5.1.5 Copyright (C) 1994-2012 Lua.org, PUC-Rio > print("Hello World!") Hello World! >
Using Lua Interpreter Script Mode
Lua interpreter also works in scripted mode. Open any text editor, enter the following text and save as hello.lua
hello.lua
print ("Hello World!")
For Windows OS, open the command prompt terminal (CMD) and run the program as shown below −
C:\>lua hello.lua
This will display the following output
Hello World!
To run the program from Linux terminal
$ lua hello.lua
This will display the following output
Hello World!
Using Shebang #! in Linux Scripts
In Linux, you can convert a Lua program into a self executable script. The first statement in the code should be a shebang #!. It must contain the path to Lua executable. In Linux, Lua is installed in /usr/bin directory, and the name of the executable is lua. Hence, we add this statement to hello.lua file
hello.lua
#!/usr/bin/lua print ("Hello World!")
You also need to give the file executable permission by using the chmod +x command
$ chmod +x hello.lua
Then, you can run the program with following command line −
$ ./hello.lua
This will display the following output
Hello World!
Thus, we can write and run Hello World program in Lua using the interpreter mode and script mode.
FAQs
1. Why the first program is called Hello World?
It is just a simple program to test the basic syntax and compiler/interpreter configuration of Lua programming language.
2. Installation of Lua is required to run Hello World program?
Yes. Lua installation is required to run Hello World program.
3. How do I run a Lua program without installing it?
TutorialsPoint developed an online environment where you can run your codes. You can use the Lua online compiler to run your Lua programs.
4. First Program Vs Hello World Program in Lua?
There is no difference. The first program of Lua is generally known as the Hello World program.