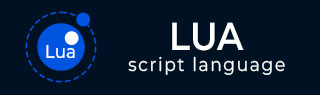
- Lua Tutorial
- Lua - Home
- Lua Basics
- Lua - Overview
- Lua - Environment
- Lua - Basic Syntax
- Lua - Comments
- Lua - Print Hello World
- Lua - Variables
- Lua - Data Types
- Lua - Operators
- Lua - Loops
- Lua - Generic For
- Lua - Decision Making
- Lua - Date and Time
- Lua Functions
- Lua - Functions
- Lua - Multiple Results
- Lua - Named Arguments
- Lua - Default/Optional Arguments
- Lua - Closures
- Lua - Uses of Closures
- Lua - Local Functions
- Lua - Anonymous Functions
- Lua - Functions in Table
- Lua - Proper Tail Calls
- Lua Strings
- Lua - Strings
- Lua - String Concatenation
- Lua - Loop Through String
- Lua - String to Int
- Lua - Split String
- Lua - Check String is NULL
- Lua Arrays
- Lua - Arrays
- Lua - Multi-dimensional Arrays
- Lua - Array Length
- Lua - Iterating Over Arrays
- Lua - Slicing Arrays
- Lua - Sorting Arrays
- Lua - Merging Arrays
- Lua - Sparse Arrays
- Lua - Searching Arrays
- Lua - Resizing Arrays
- Lua - Array to String Conversion
- Lua - Array as Stack
- Lua - Array as Queue
- Lua - Array with Metatables
- Lua - Immutable Arrays
- Lua - Shuffling Arrays
- Lua Iterators
- Lua - Iterators
- Lua - Stateless Iterators
- Lua - Stateful Iterators
- Lua - Built-in Iterators
- Lua - Custom Iterators
- Lua - Iterator Closures
- Lua - Infinite Iterators
- Lua - File Iterators
- Lua - Table Iterators
- Lua - Numeric Iterators
- Lua - Reverse Iterators
- Lua - Filter Iterators
- Lua - Range Iterators
- Lua - Chaining Iterators
- Lua Tables
- Lua - Tables
- Lua - Tables as Arrays
- Lua - Tables as Dictionaries
- Lua - Tables as Sets
- Lua - Table Length
- Lua - Table Iteration
- Lua - Table Constructors
- Lua - Loop through Table
- Lua - Merge Tables
- Lua - Nested Tables
- Lua - Accessing Table Fields
- Lua - Copy Table by Value
- Lua - Get Entries from Table
- Lua - Table Metatables
- Lua - Tables as Objects
- Lua - Table Inheritance
- Lua - Table Cloning
- Lua - Table Sorting
- Lua - Table Searching
- Lua - Table Serialization
- Lua - Weak Tables
- Lua - Table Memory Management
- Lua - Tables as Stacks
- Lua - Tables as Queues
- Lua - Sparse Tables
- Lua Lists
- Lua - Lists
- Lua - Inserting Elements into Lists
- Lua - Removing Elements from Lists
- Lua - Iterating Over Lists
- Lua - Reverse Iterating Over Lists
- Lua - Accessing List Elements
- Lua - Modifying List Elements
- Lua - List Length
- Lua - Concatenate Lists
- Lua - Slicing Lists
- Lua - Sorting Lists
- Lua - Reversing Lists
- Lua - Searching in Lists
- Lua - Shuffling List
- Lua - Multi-dimensional Lists
- Lua - Sparse Lists
- Lua - Lists as Stacks
- Lua - Lists as Queues
- Lua - Functional Operations on Lists
- Lua - Immutable Lists
- Lua - List Serialization
- Lua - Metatables with Lists
- Lua Modules
- Lua - Modules
- Lua - Returning Functions from Modules
- Lua - Returning Functions Table from Modules
- Lua - Module Scope
- Lua - SubModule
- Lua - Module Caching
- Lua - Custom Module Loaders
- Lua - Namespaces
- Lua - Singleton Modules
- Lua - Sharing State Between Modules
- Lua - Module Versioning
- Lua Metatables
- Lua - Metatables
- Lua - Chaining Metatables
- Lua - Proxy Tables with Metatables
- Lua - Use Cases for Proxy Table
- Lua - Delegation and Tracing via Proxy Tables
- Lua - Metatables vs Metamethods
- Lua - Fallback Mechanisms in Metatables
- Lua - Fallback Cases for Indexing Metamethods
- Lua - Fallback Cases for Arithmetic and Comparison Metamethods
- Lua - Fallback Cases for Other Metamethods
- Lua - Customizing Behavior with Metatables
- Lua - Controlling Table Access
- Lua - Overloading Operators
- Lua - Customizing Comparisons
- Lua - Making a Table Callable
- Lua - Customizing String Representation
- Lua - Controlling Metatable Access
- Lua Coroutines
- Lua - Coroutines
- Lua - Coroutine Lifecycle
- Lua - Communication Between Coroutines
- Lua - Coroutines vs Threads
- Lua - Chaining Coroutines
- Lua - Chaining Coroutines With Scheduler
- Lua - Chaining Coroutines Using Queues
- Lua - Coroutine Control Flow
- Lua - Nested Coroutines
- Lua File Handling
- Lua - File I/O
- Lua - Opening Files
- Lua - Modes for File Access
- Lua - Reading Files
- Lua - Writing Files
- Lua - Closing Files
- Lua - Renaming Files
- Lua - Deleting Files
- Lua - File Buffers and Flushing
- Lua - Reading Files Line by Line
- Lua - Binary File Handling
- Lua - File Positioning
- Lua - Appending to Files
- Lua - Error Handling in File Operations
- Lua - Checking if File exists
- Lua - Checking if File is Readable
- Lua - Checking if File is Writable
- Lua - Checking if File is ReadOnly
- Lua - File Descriptors
- Lua - Creating Temporary Files
- Lua - File Iterators
- Lua - Working with Large Files
- Lua Advanced
- Lua - Error Handling
- Lua - Debugging
- Lua - Garbage Collection
- Lua - Object Oriented
- Lua - Web Programming
- Lua - Database Access
- Lua - Game Programing
- Sorting Algorithms
- Lua - Bubble Sort
- Lua - Insertion Sort
- Lua - Selection Sort
- Lua - Merge Sort
- Lua - Quick Sort
- Searching Algorithms
- Lua - Linear Search
- Lua - Binary Search
- Lua - Jump Search
- Lua - Interpolation Search
- Regular Expression
- Lua - Pattern Matching
- Lua - string.find() method
- Lua - string.gmatch() method
- Lua - string.gsub() method
- Lua Useful Resources
- Lua - Quick Guide
- Lua - Useful Resources
- Lua - Discussion
Lua - Modifying List Elements
We can access the list element while traversing them and as tables are references, the modification done will reflect. In the similar fashion, after accessing the first and last element we can modify them.
We'll build the list and then add method to get first element, last element and to iterate the list and in this process, we'll update and print the values of the list.
Step 1: Create List
Create a List with a push method to add an element to the end of the list.
-- List Implementation list = {} list.__index = list -- push an element to the end of the list function list:push(t) -- move till last node if self.last then self.last._next = t t._prev = self.last self.last = t else -- set the node as first node self.first = t self.last = t end -- increment the length of the list self.length = self.length + 1 end
Step 2: Using setmetatable
modify list behavior when list is called to push elements.
setmetatable(list, { __call = function(_, ...) local t = setmetatable({ length = 0 }, list) for _, v in ipairs{...} do t:push(v) end return t end })
Step 3: Get first element and then we can modify it.
Create a function to get first element
function list:getFirst() -- if first is nil then return if not self.first then return end return self.first end
Step 4: Get Last element and then we can modify it.
Create a function to get last element
function list:getLast() -- if last is nil then return if not self.last then return end return self.last end
Step 5: Create iterator over list and update complete list during iteration.
Create an iterator to navigate through elements of the list.
-- iterate through the list local function iterate(self, current) -- if current is nil -- set the current as first node if not current then current = self.first -- if current is present -- set current as current next elseif current then current = current._next end -- return current return current end -- return the iterator function list:iterate() return iterate, self, nil end
Step 6: Test Modification of elements on List
In list, we can insert objects,
-- create a new list with values local l = list({ "Mon" }, { "Tue" }, { "Wed" }, { "Thu" }, { "Fri" }) print("Update List to make each entry in Uppercase") -- iterate throgh entries for v in l:iterate() do print(string.upper(v[1])) end -- modify first element l:getFirst()[1] = "MONDAY" print("First Element: " , l:getFirst()[1]) -- modify last element l:getLast()[1] = "FRIDAY" print("Last Element: " , l:getLast()[1])
Complete Example - Modify an element of a List
Following is the complete example of modifying elements of a list.
main.lua
-- List Implementation list = {} list.__index = list setmetatable(list, { __call = function(_, ...) local t = setmetatable({ length = 0 }, list) for _, v in ipairs{...} do t:push(v) end return t end }) -- push an element to the end of the list function list:push(t) -- move till last node if self.last then self.last._next = t t._prev = self.last self.last = t else -- set the node as first node self.first = t self.last = t end -- increment the length of the list self.length = self.length + 1 end -- iterate through the list local function iterate(self, current) if not current then current = self.first elseif current then current = current._next end return current end function list:iterate() return iterate, self, nil end function list:getLast() -- if last is nil then return if not self.last then return end return self.last end function list:getFirst() -- if first is nil then return if not self.first then return end return self.first end -- create a new list with values local l = list({ "Mon" }, { "Tue" }, { "Wed" }, { "Thu" }, { "Fri" }) print("Update List to make each entry in Uppercase") -- iterate throgh entries for v in l:iterate() do print(string.upper(v[1])) end -- modify first element l:getFirst()[1] = "MONDAY" print("First Element: " , l:getFirst()[1]) -- modify last element l:getLast()[1] = "FRIDAY" print("Last Element: " , l:getLast()[1])
Output
When we run the above code, we will get the following output−
Update List to make each entry in Uppercase MON TUE WED THU FRI First Element: MONDAY Last Element: FRIDAY